Advanced git Tactics
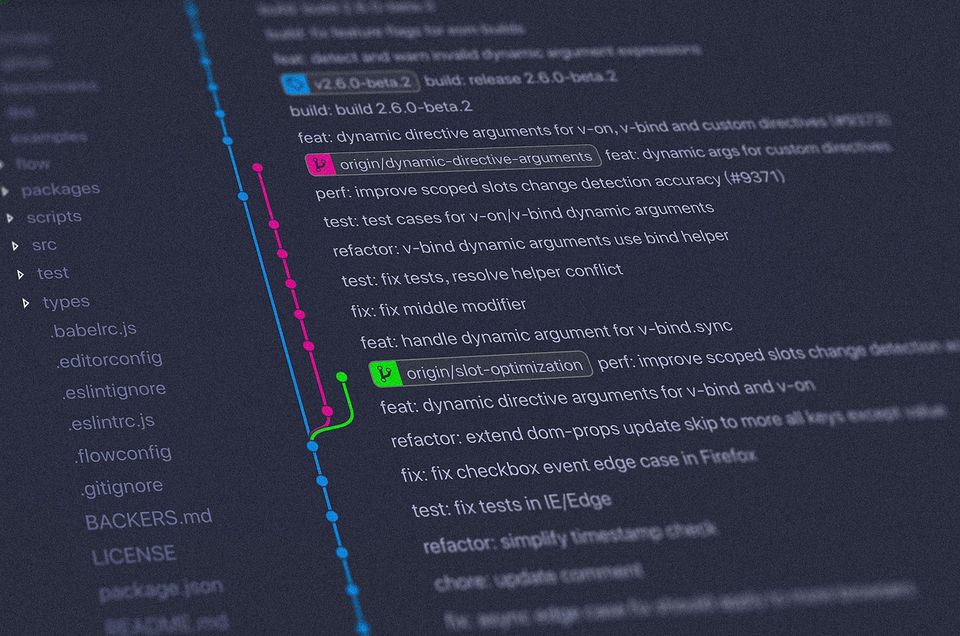
Git is an essential tool for developers, offering powerful source code management with its branching capabilities and distributed architecture. While the basics are sufficient to begin, true mastery involves advanced tactics.
This article delves into next-level strategies to elevate your Git skills. Geared towards experienced developers familiar with Git fundamentals, it covers optimizing commit history, advanced branching for complex workflows, collaboration techniques, clean resolution of merge conflicts, Git hooks for automation, bug identification through bisecting, and more. Best practices for professional Git usage, including proven methodologies to avoid pitfalls, are also highlighted.
By the end, you'll possess a deeper understanding of Git, equipped with an expert-level toolkit to handle any version control challenge. These advanced tactics will reshape your approach to Git, establishing you as a skilled practitioner. Whether managing large projects or smaller repositories, these techniques instill greater confidence and control. Let's dive in and elevate your Git skills!
Git Branching Strategies
Branching strategies form the backbone of efficient Git collaboration and organization. They define how developers work on parallel tasks, isolate changes, and integrate them into the main codebase. Choosing the right strategy for your project can vastly impact its development flow and code stability.
This section delves into three popular branching strategies, equipping you with the knowledge to make informed decisions for your specific needs:
Feature Branching
Feature branching is a straightforward workflow that thrives for small to medium sized projects. With this approach, developers create separate branches to work on new features and bug fixes in isolation. These branches allow experimentation and changes without disrupting the main codebase. Once the work is complete, the feature branch gets merged back into the integration branch through a pull request, enabling teams to track progress. Feature branching empowers developers to work independently while maintaining a clear history of changes.
# Create a new feature branch
git checkout -b feature/new-login
# Do all your work in this branch, commit and push the changes
# When done, check out the main branch
git checkout main
# Merge the feature branch back into main
git merge feature/new-login
Gitflow
For larger projects with planned releases, Gitflow offers a more structured branching strategy. Gitflow introduces additional long-running branches beyond just main and develop. These include release branches to prepare for production deployments, hotfix branches to patch urgent bugs in production, and support branches for older releases still under maintenance. The separation of concerns provided by these branches ensures stability in the critical main branch while still providing the necessary spaces for teams to work on specific tasks in parallel. However, Gitflow can also introduce complexity that may be unnecessary for some teams.
Gitflow is typically implemented using a separate Git extension tool called git-flow
. After installing, run git flow init
to initialize a Git repository for Gitflow. Afterwards you can use various git flow
commands to accomplish the desired outcome. For example:
git flow release start <release>
: Starts a release branch namedrelease/<release>
from thedevelop
branch.git flow release finish <release>
: Completes a release branch, merging it into bothdevelop
andmaster
, tagging the release, and deleting the branch.
Trunk-Based Development
Trunk-based development takes a different approach, thriving when teams prioritize continuous integration and delivery of changes. With this workflow, all development happens directly on the main branch instead of feature branches. To maintain stability, teams rely on pull request reviews, automated testing, and reverting problematic changes through hard resets.
The main advantage of trunk-based development is high visibility and collaboration since every change gets integrated immediately into the main line of development. However, this approach demands stringent code review and testing practices to avoid introducing bugs or breaking changes.
# Push directly to the main branch
git push origin main
# If ever you need to rever the changes,
# Find the SHA hash of the commit that needs to be reverted by running
git log
# Revert the problematic commit
git revert <commit-hash>
Best Practices for Git Branching
Regardless of the specific workflow, teams should follow certain best practices when leveraging Git branches:
- Use clear, descriptive naming schemes for branches to communicate their purpose
- Keep long-lived branches synchronized regularly across all developer repositories
- Establish policies to prune inactive or stale branches over time to avoid clutter
- For large features or changes, use topic branches as a way to split work into logical chunks that can be reviewed and merged incrementally
- Utilize hotfix branches to patch production issues while minimizing disruption to main development lines
- Choose workflows that suit your team's specific size, culture, and integration needs
- Master rebasing techniques to maintain a clean, linear commit history in feature branches
Key Considerations for Choosing a Branching Strategy
When evaluating branching strategies, consider factors like:
- Project size and complexity
- Team size and collaboration requirements
- Release cycles and integration frequency
- Overall development flow and code stability needs
- Ease of understanding for those new to the codebase
Commit Optimization
While Git tracks changes at a granular level, commits represent logical units of work for other developers to understand. Well-structured commits and clean history keep repositories coherent.
- Strive for commits that are atomic - making one logical change without including unrelated modifications. For example, fixing a bug and updating documentation warrant separate commits.
- Leverage Git's interactive rebase to tidy up history by squashing redundant or unclear commits. However, avoid squashing for important milestones like integrating a completed feature.
- Craft descriptive commit messages explaining the why over the how. Summarize changes in the first line, using the body for context, references, etc. Adopting conventions like prefixes (FEAT: Added login) makes scanning history easier.
- For significant changes, split into a series of focused commits telling a clear story. Commit often in local repositories, then clean up before pushing. Amend minor changes into the previous commit to avoid noisy history.
- Signing commits associates them with your identity and attests to your contribution. Use GPG keys and services like Keybase to implement signed commits in your workflow.
- Git visualization tools like GitHub's commit graph or Sourcetree offer a clear and intuitive way to understand your project's history. They provide visual representations of branches, merges, and commit messages, making it easier to see the relationships between changes.
Well-structured commits optimized through practices like rebasing, amending, and splitting changes into logical units keep repositories coherent and promote clarity. Conventional commits are a great strategy for optimizing commit history:
- Standardizes commit messages around specific prefixes like "fix" or "feat"
- Communicates the nature of changes at a glance. E.g. "fix: resolve login error when password expires"
- Enables auto-generated changelog creation
- Popular standards include Conventional Commits and Angular Commit Message Conventions
- Some use emoji prefixes for quick visual cues. E.g. "🐛 fixed issue with file upload"
Relevant git commands for commits:
git commit -m "Concise message" # commit changes with commit message.
git commit -S -m "Signed commit message" # for signing the commit.
git rebase -i <commit-hash> # to tidy up history using rebase.
git rebase -i HEAD~5 # interactive rebase to edit last 5 commits
git commit --amend # amend changes to previous commit
How to Resolve Conflicts
Merge conflicts arise when Git cannot automatically reconcile divergent changes. Learning strategies to smoothly resolve conflicts is key for any Git workflow.
Merge conflicts occur when Git encounters conflicting changes to the same file from different branches. The conflicted file is marked with special markers ("<<<<<<<", "========", ">>>>>>>") highlighting the areas of disagreement. Your job is to review these sections, choose the desired changes, and resolve the conflict manually.
Strategies for Victory:
- Manual Merge: For straightforward conflicts, this tried-and-true method involves opening the conflicted file in a text editor and combining the desired changes from both branches. Consider factors like functionality, clarity, and code style when making your decisions.
- Visual Merge Tools: Powerful tools like KDiff3 or Meld present the conflicting changes side-by-side, allowing you to visually compare and select them. Ideal for beginners or complex conflicts.
- Interactive Rebase: In some cases, you can use interactive rebasing to rewrite history and avoid merge conflicts altogether. However, proceed with caution, as this rewrites history and can affect team collaboration.
The Keys to Success:
- Calm and Clarity: Approach merge conflicts with a clear mind and a patient heart. Haste can lead to poor decisions and further complications.
- Communication is Key: If collaborating with others, keep them informed about the conflict resolution process and seek input if needed. Clear communication ensures everyone remains on the same page.
- Pull latest upstream changes frequently: You can avoid many conflicts by frequently pulling latest upstream changes, dealing with conflicts locally, and keeping commits and branches small and focused. Rebasing local work onto the updated upstream often yields a smoother merge.
- Learning from Experience: Each merge conflict presents an opportunity to learn and improve your Git skills. Analyze the causes of the conflict and explore ways to prevent them in the future.
With the right strategies and tools, conflict resolution becomes a manageable part of your workflow instead of a frustrating impediment. Mastering these techniques will enable you to smoothly integrate parallel work.
Useful git commands to employ when merging changes and resolving conflicts:
git merge branch-with-conflicts # merge a branch with conflicts
git mergetool # launch merge tool to resolve conflicts
git rebase master # rebase current branch onto master
Collaboration and Sharing
Git facilitates collaboration through robust tools for sharing and syncing repositories. Mastering these collaborative workflows unlocks Git's full potential for teamwork.
- Manage contributions from many developers via pull requests on GitHub, GitLab, or Bitbucket. Use built-in review tools to discuss and refine changes before merging.
- Keep local repositories in sync with GitHub's forking workflow. Configure remotes to pull upstream changes from the original repo into your fork.
- Enable continuous integration by connecting Git repositories to services like Travis CI or CircleCI. Automate building, testing, and deployment based on Git events like new commits.
- Publish public package repositories with Git servers like GitHub Package Registry. Link your project's dependencies to hosted Git repositories.
- Share code snippets or gists without a full repository. Paste code into GitHub gists to share and embed code blocks.
- For repositories with external dependencies, use Git submodules. Submodules point to a specific commit in another respository to reference it.
- Follow upstream changes in a vendor branch with remote tracking branches. Periodically merge upstream vendor branches into your local work.
With robust collaboration capabilities like forking, pull requests, and submodules, Git allows teams to coordinate changes across repositories. These shared workflows enable powerful new Git-based collaboration paradigms.
Relevant git commands:
git remote add upstream <url> # Add remote named upstream
git pull upstream master # Pull latest from upstream master
git push origin my-feature # Push feature branch to origin
Monorepo vs Multi-Repo vs Git Submodules
Monorepo
With a monorepo approach, all project code lives together in a single repository. This simplifies dependency management between components since everything is in one place. Atomic changes across modules are also easier since the entire codebase is versioned together.
However, monorepos can become large and unwieldy without proper organization. Code needs to be logically segmented within the repository, often using nested subfolders or libraries. Strict conventions around commit hygiene and code isolation must be followed at scale. Overall, monorepos thrive for highly interconnected systems with shared ownership where atomic changes are frequent.
Multi-Repo
In contrast, a multi-repo structure segments code into multiple dedicated repositories. This isolates unrelated code into conceptual groups that are easier to manage. However, it does add complexity in managing dependencies across repositories. Duplicate and inconsistent tooling can also emerge when each repository functions independently.
Strong conventions around repository naming, API contracts, and dependency versioning become essential. Generally, independent systems with distinct functions or ownership benefit most from being separated into their own repositories.
Git Submodules
Finally, Git submodules provide a hybrid approach. The main project repository contains subdirectories linking to other repositories as submodules. This allows combining related projects under one umbrella while still maintaining isolation between them.
However, submodules do increase cognitive overhead and command complexity compared to monorepos. Submodule pointers are also pinned to specific commits rather than branches, complicating version management. For moderately coupled systems, submodules offer a balance between full consolidation and complete separation.
Managing External Dependencies
All three approaches can incorporate external dependencies using package managers like npm, Composer, or NuGet. These should be referenced in configuration files rather than directly in the repositories. For private packages, solutions like GitHub Package Registry or npm Private Packages provide Git-based hosting with access controls.
Git Hooks and Automation
Git hooks allow you to trigger custom scripts and workflows in response to events like committing or pushing code. Hooks help automate repetitive tasks and enforce standards.
- Use pre-commit hooks to lint, validate, or format code before allowing a commit. This saves others from reviewing bad code.
- Leverage prepare-commit-msg hooks to influence the message style and content. For example, prepend ticket numbers.
- Post-commit hooks enable workflows like pushing commits to another repository after commit.
- Implement pre-push hooks to run test suites and deny pushing if tests fail.
- Standardize commit messages with commit-msg hooks that check for required formats.
- Generate automatic documentation on push by extracting information from code comments.
- Deploy application updates automatically by connecting pushes to continuous integration tools.
- Init templates provide starter hooks for common automation scenarios like linting and testing.
Advanced Tools and Techniques
Git offers advanced tools and options for specialized workflows and troubleshooting beyond basic commits and branches.
- Git bisect quickly finds bug-introducing commits by doing a binary search through history. Isolate regressions without manual trial and error. You can use
git bisect start
to start the bisect session, andgit bisect good/bad
to find bug-introducing commits. - Git stash temporarily shelves uncommitted changes to allow switching contexts cleanly. Stash, then pop or apply stashes when ready to continue work.
git stash push
can be used to temporarily shelve changes. - Git subtrees allow managing nested git repositories together while still isolating modules. Useful for combining many repositories or component libraries.
- Git cherry-pick selectively applies commits from another branch. Port bug fixes or features between branches without full merges. Use
git cherry-pick <commit-hash>
to apply a specific commit from another branch. git reflog
tracks previous HEAD references and branch pointers, enabling undoing destructive operations like resets.- Git rerere records how merge conflicts were resolved and reuses the resolution in future merges.
Conclusion
Mastering advanced Git tactics provides tangible benefits for individual developers and engineering teams. Techniques like optimized commit hygiene, collaborative workflows, and automation set users up for efficiency, visibility, and confidence in their version control practices.
This guide explored numerous productivity-boosting Git tactics suitable for intermediate to advanced practitioners. While Git's basic commands are easy to pick up, truly unlocking its potential requires leveling up your skills. Developers comfortable with Git fundamentals can build expertise in specialized areas like Git hooks, interactive rebasing, and branching strategies.
With practice, the workflows covered here become second nature. Maintaining clean commit history, resolving tricky merge conflicts, undoing mistakes safely, and collaborating seamlessly all reduce friction during development. They position you to spend less time wrestling with version control so you can focus on building great software.
That was a summary of the discussion we had in community event last week, see you again this week for the next event on Discord.
Additional Resources
Explore more discussions on this topic and deepen your understanding with the following links:
What’s the best way to deal with or reduce the occurrence of git merge conflicts?
by u/Srr013 in AskProgramming
What are your best practices to minimize merge conflicts?
by u/tinkert00l in git
Monorepo, multirepo and Git submodules
by u/vadishev in git
How about taking this discussion one step forward and share your thoughts on this topic anonymously on Invide community forum
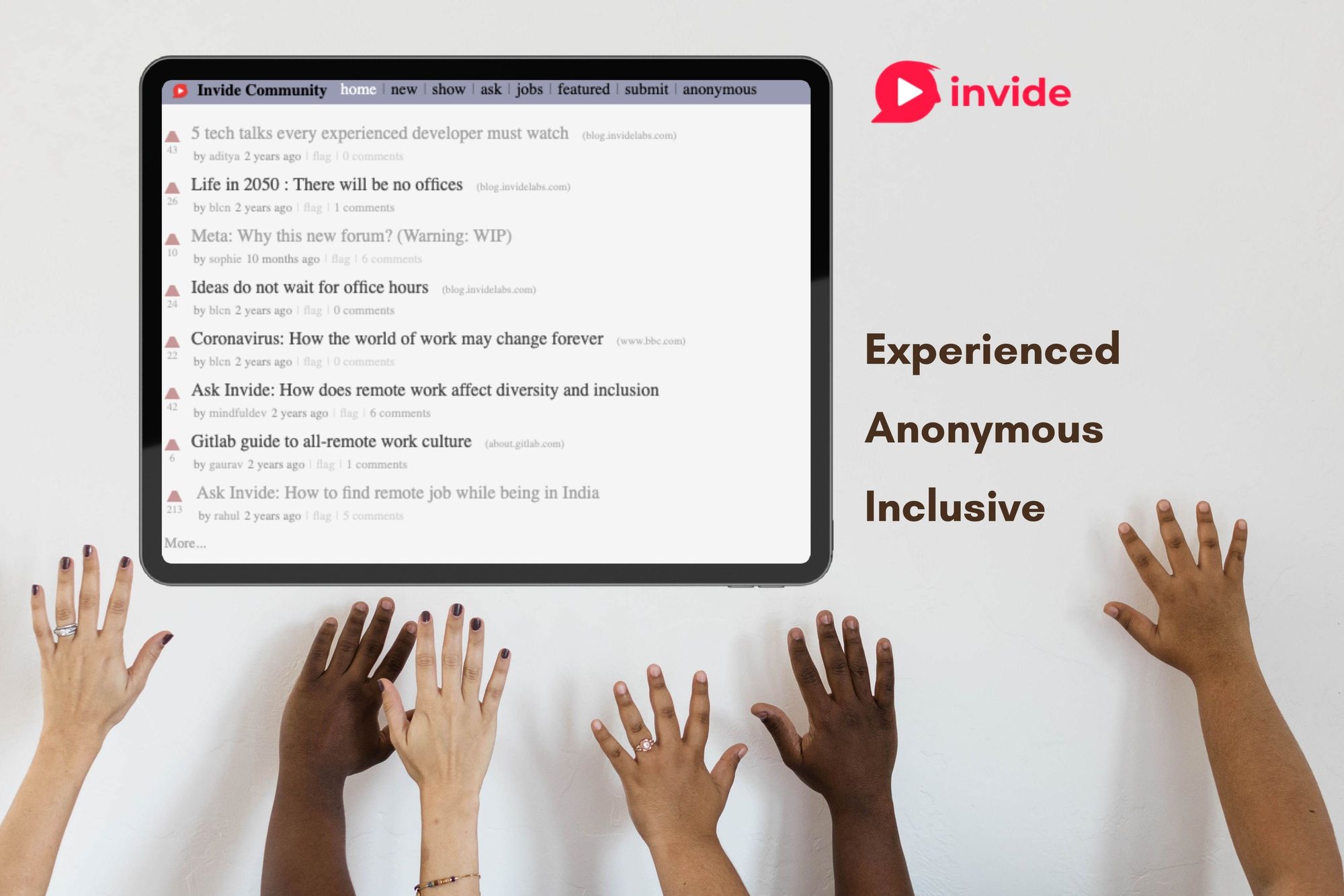
Member discussion