What is Resilient Distributed Systems (RDD)
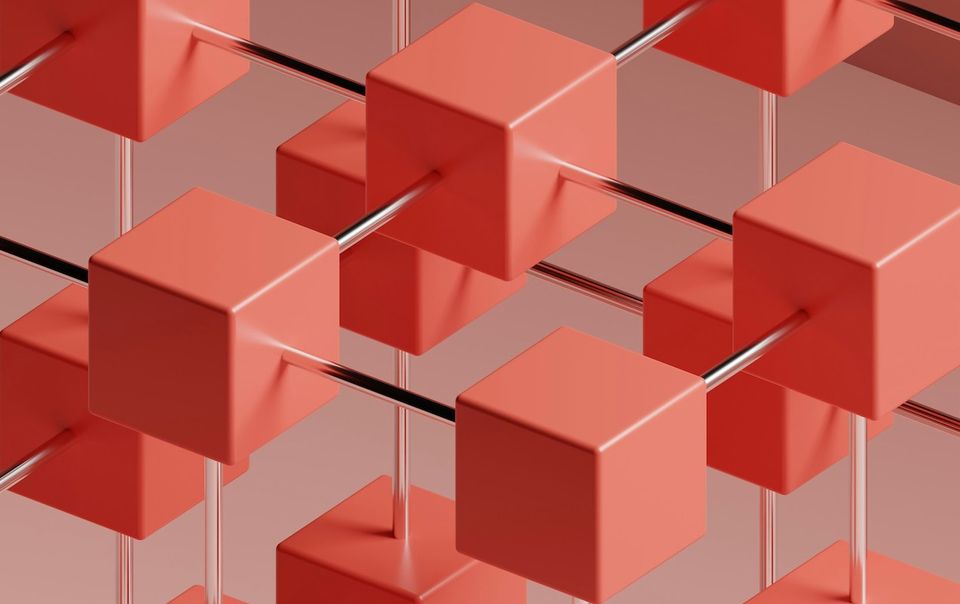
Software that survives chaos
Imagine you're building a mission-critical application that needs to handle millions of requests, survive hardware failures, and maintain data integrity—all while delivering lightning-fast performance. Welcome to the world of Resilient Distributed Systems, where complexity meets innovation and reliability is not just a feature, but a fundamental design principle.
What makes a system truly resilient?
At its core, a resilient distributed system is like a sophisticated organism—adaptable, self-healing, and capable of continuing operations even when individual components fail. It's not just about preventing failures, but gracefully managing them when they inevitably occur.
Key characteristics of resilience:
Fault Tolerance: The ability to continue operating correctly when one or more components fail. Think of it as a digital safety net that prevents total system collapse.
# Simple example of a fault-tolerant approach
def process_request(request):
try:
# Primary processing logic
result = primary_service.handle(request)
return result
except PrimaryServiceError:
# Fallback to backup service if primary fails
return backup_service.handle(request)
Horizontal Scalability: The system can grow by adding more machines to the network, rather than upgrading existing hardware. It's like adding more workers to a construction site instead of expecting superhuman performance from a single worker.
Data Consistency Models: Balancing between strong consistency (every read returns the most recent write) and eventual consistency (reads might temporarily return slightly outdated data, but will converge).
The architectural pillars of resilience
1. Distributed Consensus
Consensus algorithms like Raft and Paxos ensure that multiple nodes in a system can agree on a single data value or state, even in the face of partial failures.
// Simplified Raft consensus pseudocode
func electLeader(cluster) {
// Nodes negotiate and elect a leader
// Ensures a single source of truth
for each node in cluster:
if node.canBeLeader() && node.hasMoreUpToDateLog():
node.becomeLeader()
}
2. Microservices and Decoupling
By breaking down monolithic applications into smaller, independent services, we create systems that can heal and scale individual components without bringing down the entire ecosystem.
3. Circuit Breakers
Prevent cascading failures by temporarily disabling a service that's experiencing issues, giving it time to recover.
class CircuitBreaker {
private State state = CLOSED;
public Response executeRemoteCall() {
if (state == CLOSED) {
try {
return actualRemoteCall();
} catch (Exception e) {
recordFailure();
if (failureThresholdExceeded()) {
state = OPEN;
}
}
}
// Fail fast or return cached/default response
}
}
Real-world challenges and solutions
Handling Network Partitions
The famous "split-brain" problem occurs when network issues prevent nodes from communicating. Solutions like Quorum-based systems ensure that only a majority of nodes can make critical decisions.
Data Replication Strategies
- Primary-Backup: One node handles writes, others provide redundancy
- Multi-Primary: Multiple nodes can handle writes, with complex synchronization
- Eventual Consistency: Prioritizes availability over immediate consistency
Applications of RDD (Resilient Distributed Systems)
Cloud Computing
- Cloud platforms like Amazon Web Services (AWS), Google Cloud, and Microsoft Azure rely on resilient distributed systems to provide scalable, reliable, and fault-tolerant services. Cloud computing enables businesses to deploy applications that are resilient by design, with data stored redundantly and services spread across multiple regions for high availability.
E-Commerce
- For online retailers, uptime and performance are critical. Resilient distributed systems allow e-commerce platforms to handle millions of concurrent users and transactions, ensuring fast load times, high availability, and continuous operation during peak sales periods (e.g., Black Friday).
Content Delivery Networks (CDNs)
- CDNs distribute content across multiple servers worldwide to ensure fast access to websites and services for users, regardless of their location. These systems are resilient, automatically routing requests to the nearest available server if one server goes down.
Social Media
- Platforms like Facebook, Twitter, and Instagram rely on resilient distributed systems to serve billions of active users simultaneously. These systems handle the vast amounts of data generated by user activity, ensuring that the services remain available and responsive.
Financial Services
- In the financial industry, resilient distributed systems are used to ensure that transactions, market data feeds, and banking services are always available. High availability and fault tolerance are crucial for applications like stock trading platforms or payment processing systems.
Tools of the trade
- Apache Kafka: Distributed streaming platform
- Kubernetes: Container orchestration
- Zookeeper: Distributed coordination service
- etcd: Distributed key-value store
Conclusion - Designing with the human element (failure) in mind
Resilience is as much a mindset as it is a technical implementation. As developers, we must:
- Anticipate failures
- Design for graceful degradation
- Implement comprehensive monitoring
- Create robust fallback mechanisms
By understanding and implementing these principles, you're not just writing code; you're architecting digital ecosystems that can survive and thrive in the most challenging environments.
In the world of distributed systems, failure is not an exception—it's the rule. Your job is to make sure that when things fall apart, your system knows how to put itself back together.
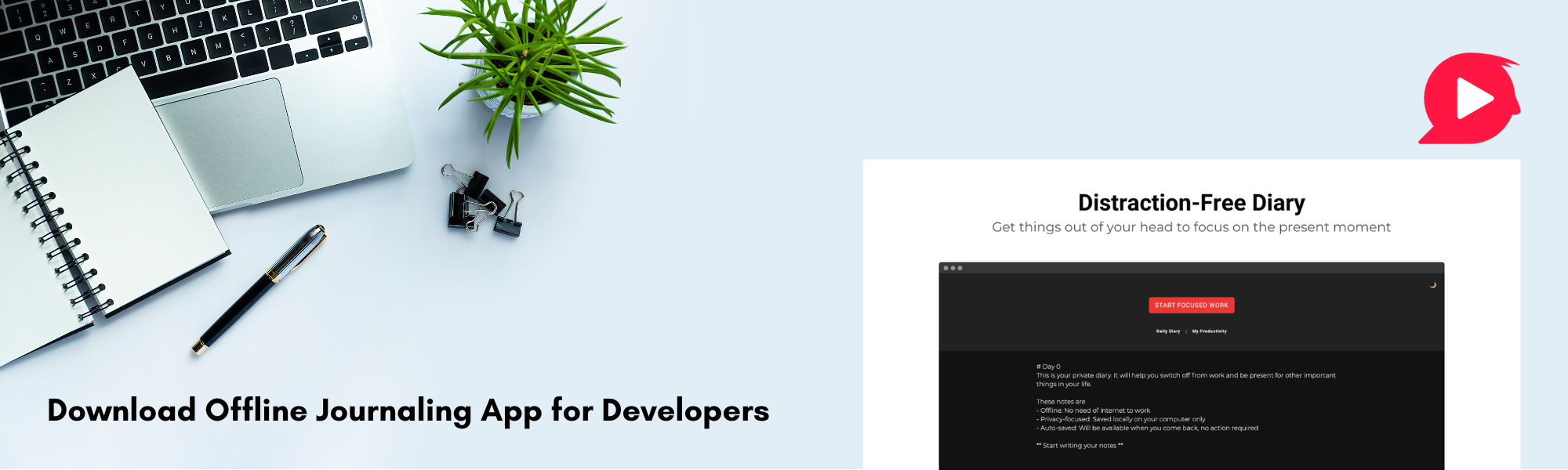
Member discussion